LeetCode每日一题
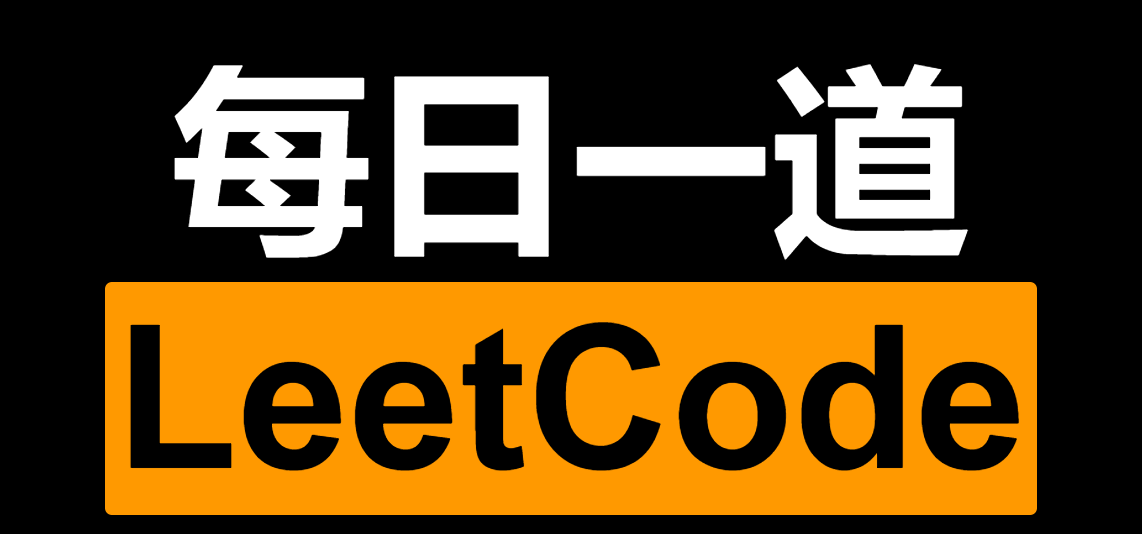
LeetCode每日一题
sinarcsinxLeetCode每日一题
✨2024-12-27
📙1. 两数之和
法一:遍历数组
1 | public int[] twoSum(int[] nums, int target) { |
法二:利用哈希表
num
为key
index
为value
1 | public int[] twoSum(int[] nums, int target) { |
📙175. 组合两个表
法一:左联表
1 | # Write your MySQL query statement below |
✨2024-12-28
📙2. 两数相加
法一:合并两数且遍历
1 | public ListNode addTwoNumbers(ListNode l1, ListNode l2) { |
📙176. 第二高薪水
法一:排除最高薪水再次搜索最大薪水
1 | # Write your MySQL query statement below |
法二:排序后取第二高薪水
1 | # Write your MySQL query statement below |
✨2024-12-29
📙3. 无重复字符的最长字串
法一:通过HashSet判断
1 | public int lengthOfLongestSubstring(String s) { |
📙177. 第N高薪水
法一:排序后获取第N高薪水
1 | CREATE FUNCTION getNthHighestSalary(N INT) RETURNS INT |
✨2024-12-30
📙4. 寻找两个正序数组的中位数
法一:合并并排序后找中位数
1 | public double findMedianSortedArrays(int[] nums1, int[] nums2) { |
📙178. 分数排名
法一:使用dense_rank()排序
1 | # Write your MySQL query statement below |
收获:
函数 | 相同值处理 | 排名跳跃 |
---|---|---|
ROW_NUMBER() |
每行唯一编号 | 无 |
RANK() |
相同值相同排名,后续跳过 | 有 |
DENSE_RANK() |
相同值相同排名,后续不跳过 | 无 |
NTILE(n) |
将数据划分为 n 组 | 无 |
✨2024-12-31
📙5. 最长回文子串
法一:从中间向两边遍历
1 | public String longestPalindrome(String s) { |
📙180. 连续出现的数字
法一:内连接
1 | # Write your MySQL query statement below |
✨2025-1-1
📙6. Z字形变换
法一:根据字形形成的数据创建集合
1 | public String convert(String s, int numRows) { |
📙181. 超过经理收入的员工
法一:连表查询
1 | # Write your MySQL query statement below |
法二:子查询
1 | # Write your MySQL query statement below |
✨2025-1-2
📙7. 整数反转
法一:移动尾数
1 | public int reverse(int x) { |
📙182. 查找重复的电子邮箱
法一:使用Having,group by
1 | # Write your MySQL query statement below |
✨2025-1-3
📙8. 字符串转换整数 (atoi)
法一:去空格后遍历,之后判断是否超出范围
1 | public int myAtoi(String s) { |
📙183. 从不订购的用户
法一:子查询
1 | # Write your MySQL query statement below |
法二:连表查询
1 | # Write your MySQL query statement below |
✨2025-1-4
📙9. 回文数
法一:转换为字符数组,左右对比
1 | public boolean isPalindrome(int x) { |
法二:获取原数的回文数,判断两数是否相同
1 | public boolean isPalindrome(int x) { |
📙184. 部门工资最高的员工
法一:连表查询
1 | # Write your MySQL query statement below |
1 | # Write your MySQL query statement below |
✨2025-1-5
📙13. 罗马数字转整数
法一:前数大于后数则加,反之则减
1 | public int romanToInt(String s) { |
📙185. 部门工资前三高的所有员工
法一:连表查询
1 | # Write your MySQL query statement below |
✨2025-1-6
📙12. 整数转罗马数字
法一:罗列数字,从大到小转换
1 | public String intToRoman(int num) { |
📙196. 删除重复的电子邮箱
法一:子查询
1 | # Write your MySQL query statement below |
✨2025-1-7
📙19. 删除链表的倒数第 N 个结点
法一:遍历找到结点总数,再找倒数第n个节点
1 | public ListNode removeNthFromEnd(ListNode head, int n) { |
📙197. 上升的温度
法一:连表查询
1 | # Write your MySQL query statement below |
✨2025-1-8
📙20. 有效的括号
法一:使用栈
1 | public boolean isValid(String s) { |
📙511. 游戏玩法分析 I
法一:子查询
1 | # Write your MySQL query statement below |
✨2025-1-9
📙21. 合并两个有序链表
法一:遍历两个链表,依次加入
1 | public ListNode mergeTwoLists(ListNode list1, ListNode list2) { |
📙577. 员工奖金
法一:连表查询
1 | # Write your MySQL query statement below |
✨2025-1-10
📙26. 删除有序数组中的重复项
法一:遍历,通过计数改变原有项
1 | public int removeDuplicates(int[] nums) { |
📙584. 寻找用户推荐人
法一:is null
1 | # Write your MySQL query statement below |
✨2025-1-11
📙27. 移除元素
法一:遍历删除
1 | public int removeElement(int[] nums, int val) { |
📙570. 至少有5名直接下属的经理
法一:连表查询
1 | # Write your MySQL query statement below |
✨2025-1-12
📙28. 找出字符串中第一个匹配项的下标
法一:遍历
1 | public int strStr(String haystack, String needle) { |
优化:
1 | public int strStr(String haystack, String needle) { |
📙586. 订单最多的客户
法一:子查询
1 | # Write your MySQL query statement below |
法二:普通查询
1 | # Write your MySQL query statement below |
✨2025-1-13
📙35. 搜索插入位置
法一:二分查找
1 | public int searchInsert(int[] nums, int target) { |
📙595. 大的国家
法一:普通查找
1 | # Write your MySQL query statement below |
✨2025-1-14
📙58. 最后一个单词的长度
法一:遍历计数
1 | public int lengthOfLastWord(String s) { |
📙596. 超过5名学生的课
法一:普通查找
1 | # Write your MySQL query statement below |
✨2025-1-15
📙53. 最大子数组和
法一:动态规划
1 | public int maxSubArray(int[] nums) { |
📙607. 销售员
法一:嵌套查询
1 | # Write your MySQL query statement below |
✨2025-1-16
📙54. 螺旋矩阵
法一:设置4个标识后按顺序遍历
1 | public List<Integer> spiralOrder(int[][] matrix) { |
📙610. 判断三角形
法一:使用if判断
1 | # Write your MySQL query statement below |
✨2025-1-17
📙59. 螺旋矩阵 II
法一:同螺旋矩阵,设置标识后遍历
1 | public int[][] generateMatrix(int n) { |
📙619. 只出现一次的最大数字
法一:子查询
1 | # Write your MySQL query statement below |
✨2025-1-18
📙61. 旋转链表
法一:将单向连表变为螺旋链表再切断
1 | public ListNode rotateRight(ListNode head, int k) { |
📙620. 有趣的电影
法一:普通查找
1 | # Write your MySQL query statement below |
✨2025-1-19
📙66. 加一
法一:加一后遍历,如果最高位加一则新建数组
1 | public int[] plusOne(int[] digits) { |
📙627. 变更性别
法一:使用条件判断语句
1 | # Write your MySQL query statement below |
✨2025-1-20
📙67. 二进制求和
法一:首部不够则添0,遍历
1 | public String addBinary(String a, String b) { |
📙1050. 合作过至少三次的演员和导演
法一:通过 group 普通查找
1 | # Write your MySQL query statement below |
✨2025-1-21
📙69. x 的平方根
法一:袖珍计算器算法
1 | public int mySqrt(int x) { |
📙1068. 产品销售分析 I
法一:连表查询
1 | # Write your MySQL query statement below |
✨2025-1-22
📙73. 矩阵置零
法一:遍历存位置
1 | public void setZeroes(int[][] matrix) { |
优化空间复杂度:
1 | public void setZeroes(int[][] matrix) { |
优化遍历方法:
1 | public void setZeroes(int[][] matrix) { |
📙1075. 项目员工 I
法一:通过聚合函数计算
1 | # Write your MySQL query statement below |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果